In this blog post we will crate a set of actions which will allow us to run any vRA8 REST call. vRealize Automation 8 Swagger documentation can be found under url:
https://<vra-server-hostname>/automation-ui/api-docs/
You can find all vRA services here. In this example we will run a request to get all vRA deployments form Deployment service:
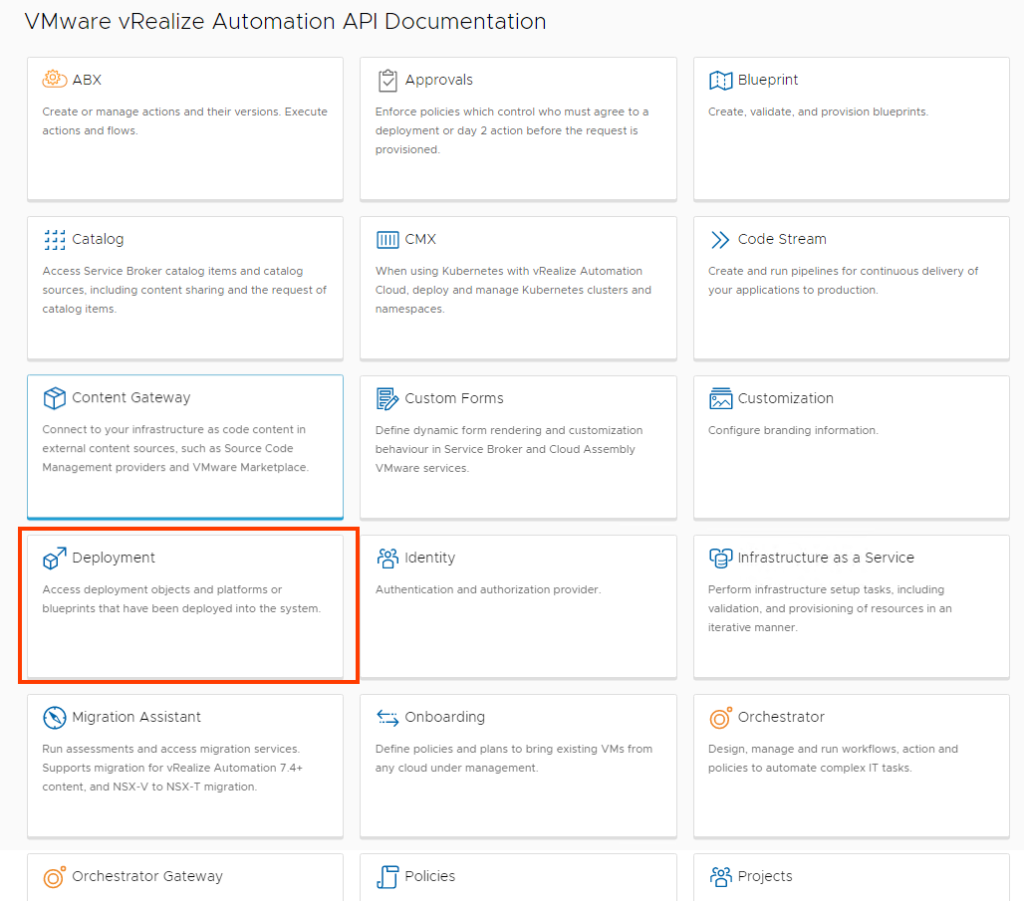
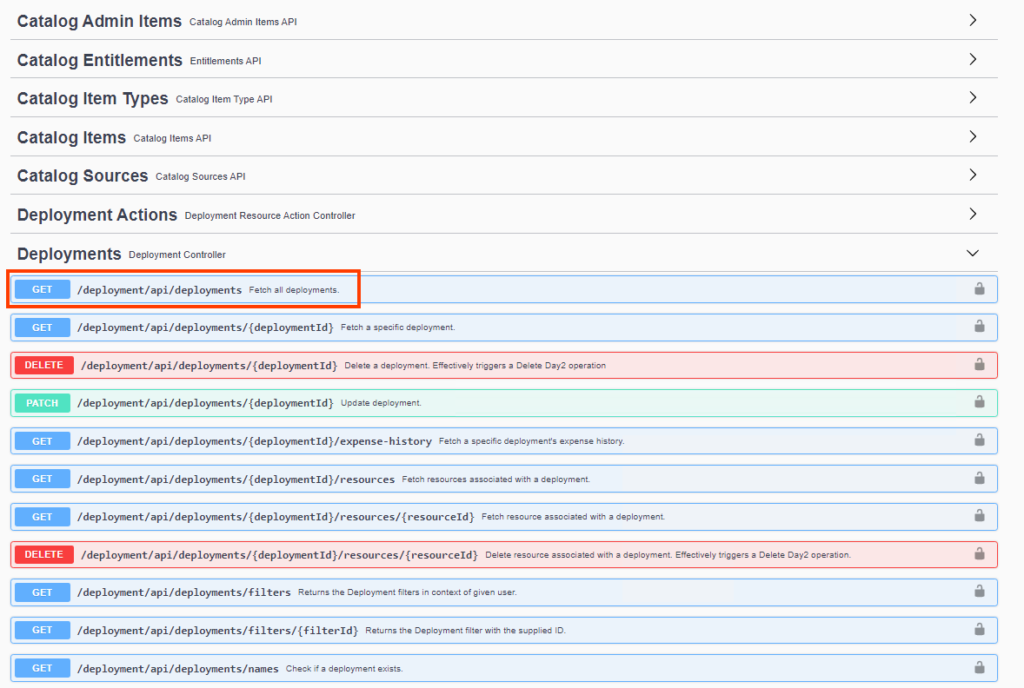
First we have to create a vRO REST endpoint for our vRA. This will allow us to run HTTP request from vRO against vRA server. To achieve this we have to run “Add a Rest host” Workflow form vRO Library. Do not set any authentication, we will have handle it manually. Once done you should be able to see new REST endpoint in vRO Inventory under HTTP-REST.
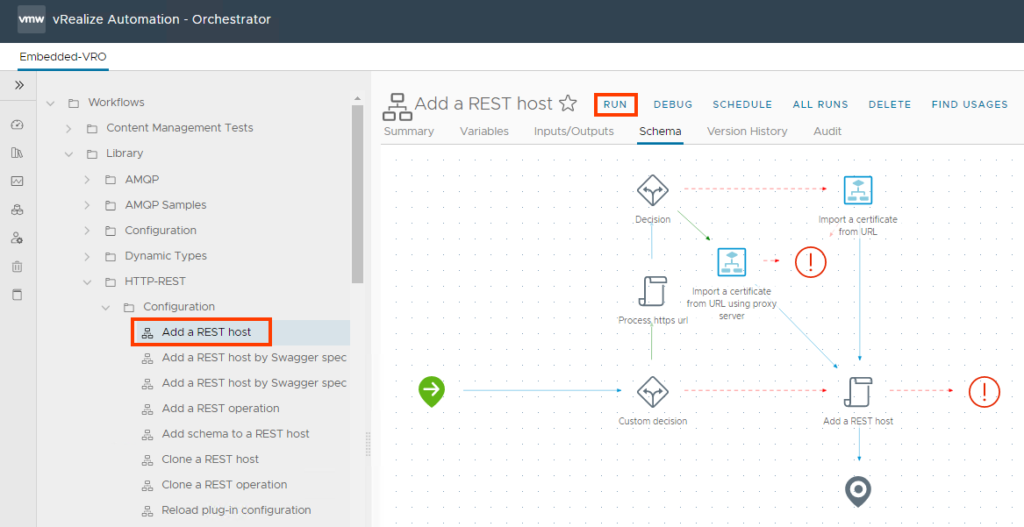
Now create new vRO Action module com.vra.rest.utils (or any name than fits your naming convention). We will create 4 new actions and fill them with content as we go. This is our end state.
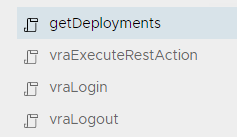
First we have to work on login action. I’m storing all endpoints in vRO config element. Then when I need it in the action I’m recovering endpoint object form this config element. You can use action input for that as well, but this way you will be able to run the action directly much easier. Especially if you want to run it from different action.
To get endpoint in the action I use getConfigElementAttributeValue() this function code is available here. You can store user name and password for vRA access in vRO config element as well and get it this same way. This way it will not be hardcoded in your actions 😉
//Params:
//<none>
//Return:String
//Get vRA access token
var restHost = System.getModule("com.configuration.utils").getConfigElementAttributeValue("Global", "Endpoinds", "vRARest");
var domain = 'domain.lab';
var user = 'vRAUser';
var password = 'P@$$w0rw';
//Login Object
var loginObj = {};
loginObj.domain = domain;
loginObj.password = password;
loginObj.scope = ""
loginObj.username = user;
var loginJason = JSON.stringify(loginObj);
//login URL
var operationUrl = "/csp/gateway/am/api/login"
var request = restHost.createRequest("POST", operationUrl, loginJason);
request.setHeader("Content-Type", "application/json");
var response = request.execute();
System.debug(response.contentAsString);
var res = JSON.parse(response.contentAsString);
var token = res.cspAuthToken;
System.log("token: " + token);
return token
Save this as varLogin, we will use it later. Remember to set return type to String. Now lets work on logout
//Params:
// - token:string - vRA access token
//Return:string
//Get vRA access tokenvar restHost = System.getModule("com.configuration.utils").getConfigElementAttributeValue("Global", "Endpoinds", "vRARest");
//Logout
var logoutObj = {};
logoutObj.idToken = token;
var logoutJson = JSON.stringify(logoutObj);
var operationUrl = "/csp/gateway/am/api/auth/logout"
var request = restHost.createRequest("POST", operationUrl, logoutJson);
request.setHeader("Content-Type", "application/json");
var response = request.execute();
return response.statusCode
Remember to set input and return type for all actions.
Once we have login and logout functions we can work on an action that will allow us to run any REST operation form Swagger documentation vRAExecuteRestAction. This function will have 3 inputs. Type of http call GET/POST/PUT…, url we want to call and if this is a post action a stringify JSON payload we want to send. This function is returning stringify payload so if you want to work with this object you will JSON.parse() it.
//Params:
// - method:string - REST metod GET/POST/PUT...
// - operationUrl:string - operation URL
// - content:string - if POST request stringify content
//Return:String
var restHost = 'vRA Host REST endpoint in vRO, Workflow attribute';
//login
var vraToken = System.getModule("com.vra.rest.utils").vraLogin();
if(content){
var request = restHost.createRequest(method, operationUrl, content);
}
else{
var request = restHost.createRequest(method, operationUrl);
}
request.setHeader("Content-Type", "application/json");
request.setHeader("Authorization", "Bearer " + vraToken);
var response = request.execute();
var statusCode = response.statusCode;
var responseContent = response.contentAsString;
if(statusCode > 399) {
System.error(responseContent);
throw "executeRestAction Failed, Status Code: " + statusCode;
}
return responseContent;
//Logout
var retCode = System.getModule("com.vra.rest.utils").vraLogout(token);
Now last piece the test if everything is working as expected. We will get a JSON representation for all deployments on our vRA. To do that we have to call GET request on “/deployment/api/deployments” url. Content of getDeployments action is simple:
var content = System.getModule("com.vra.rest.utils").vraExecuteRestAction('GET', '/deployment/api/deployments', null);
System.log(content);
var id = JSON.parse(content).content[0].id;
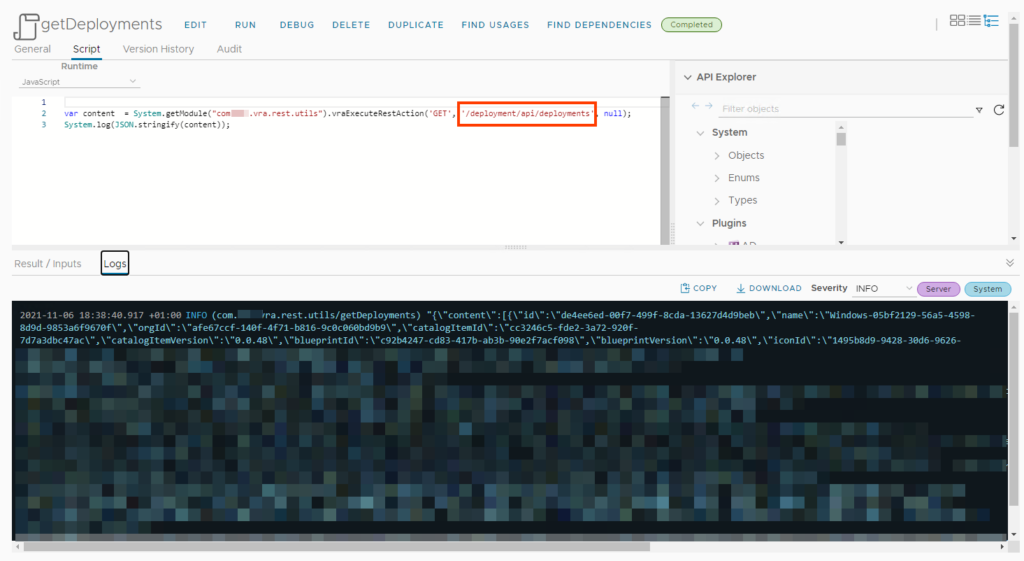
As you can see we did receive back a answer containing all deployments on our vRA instance, Now you can run any vRA Rest call good luck 😉