This is basic date manipulation library for vRO. DateJs is an JavaScript Date library for parsing and processing dates. It allows for many operations and calculations like: comparing dates, add time spans to a given date, or get N-th day of the week in a given month etc.
If you need to calculate a date for a first Wednesday in October in two year it will help you a bit I hope.
Below you will find an examples of how to initiate the library in vRO and what you can do with it:
Library usage examples:
//Init
var DateCalc = System.getModule("com.github.datejs").DateService();
var dateCalc = new DateCalc(); //now
//Add Operations
dateCalc = new DateCalc(); //now
dateCalc.add({days:1}); //tomorrow
dateCalc.add({days: -1}); //yesterday
dateCalc.add({months:1}); //next month
dateCalc.add({days:1,months:1}); // in 1 month and a day
dateCalc.add({weeks:2}); // in 2 weeks
dateCalc.add({ years: -1 }); //year before
dateCalc.addSeconds(120); // add 120 sec
dateCalc.addMinutes(2); // add 2 minutes
dateCalc.addDays(2); // add 2 days
dateCalc.addWeeks(1); // add 1 week
dateCalc.addMonths(2); //add 2 months
dateCalc.addYears(2); //add 2 years
//Set Operations
var dateCalc = new DateCalc();
dateCalc.set({minute:10});
dateCalc.set({hour:2});
dateCalc.set({day:20});
dateCalc.set({month:1});
dateCalc.add({days:1}).set({hour:12}).set({minute:00}); //tomorrow 12:00
//Compare Operations
dateCalc.compare(now, yesterday);
dateCalc.equals(yesterday, tomorrow);
dateCalc.between(yesterday, now, tomorrow));
dateCalcToday.isBefore(tomorrow);
dateCalcToday.isAfter(tomorrow);
For full list of available operations check “Date Lib Test” workflow in the package.
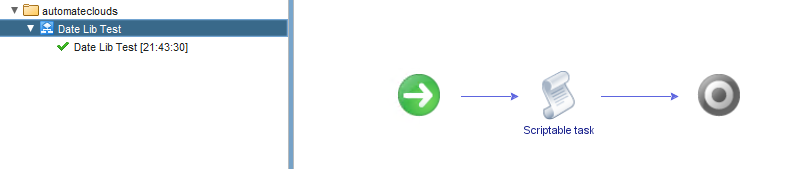