In my vRealize Orchestrator a lot of workflows are being scheduled to be executed automatically in future. Since i like keeping it clean I have a workflow scheduled to be executed daily to clean up all old executed workflows. This way my Scheduled Workflows tab in vRO is always clean.
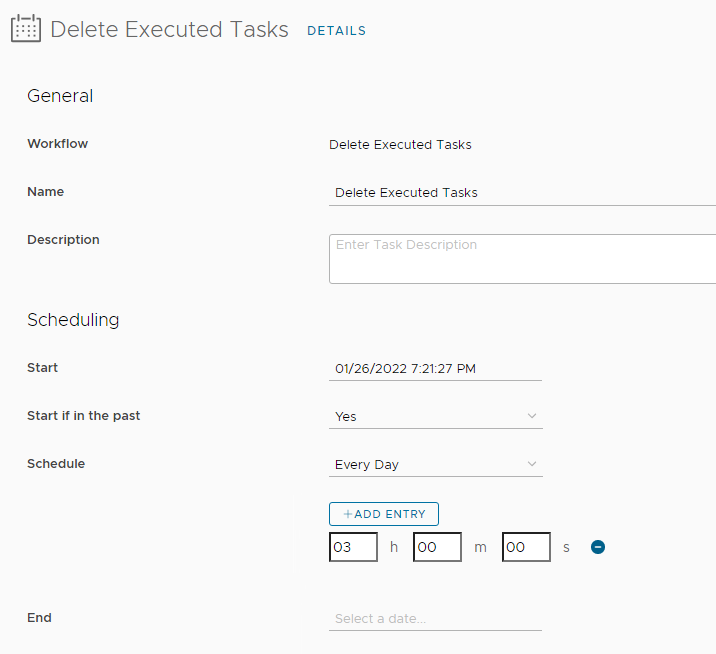
To use the code below you have to create a vRO REST endpoint with basic authentication and set it as a variable in the workflow.
/*
* INPUTS:
* vroHost <REST:RESTHost> - vRO rest endpoint with basic authentication
*/
operationUrl = "/api/tasks"
request = vroHost.createRequest("GET", operationUrl);
request.setHeader("Accept", "application/json");
request.setHeader("Content-Type", "application/json");
var response = request.execute();
if(response.statusCode >= 300){
throw 'Error while trying to execute request! response.statusCode: ' + response.statusCode;
}
else {
var response = JSON.parse(response.contentAsString);
for each (var task in response.relations.link){
var finished = false;
var oneTime = false;
for each (var attrib in task.attributes){
if (attrib.name == "recurrenceCycle"){
System.debug("recurrenceCycle: " + attrib.value);
if(attrib.value == "one-time"){
oneTime = true;
}
}
if (attrib.name == "state"){
System.debug("state: " + attrib.value);
if(attrib.value == "finished"){
finished = true;
}
}
}
var id = task.href.split("/");
id = id[id.length-2];
if (finished && oneTime){
System.warn("DELETE TASK: " + id);
deleteTask(id);
}
}
}
function deleteTask(id){
operationUrl = "/api/tasks/" + id
request = vroHost.createRequest("DELETE", operationUrl);
request.setHeader("Accept", "application/json");
request.setHeader("Content-Type", "application/json");
var response = request.execute();
if(response.statusCode >= 300){
throw 'Error while trying to execute Delete request! response.statusCode: ' + response.statusCode;
}
else {
System.debug('Delete response.statusCode: ' + response.statusCode);
}
}